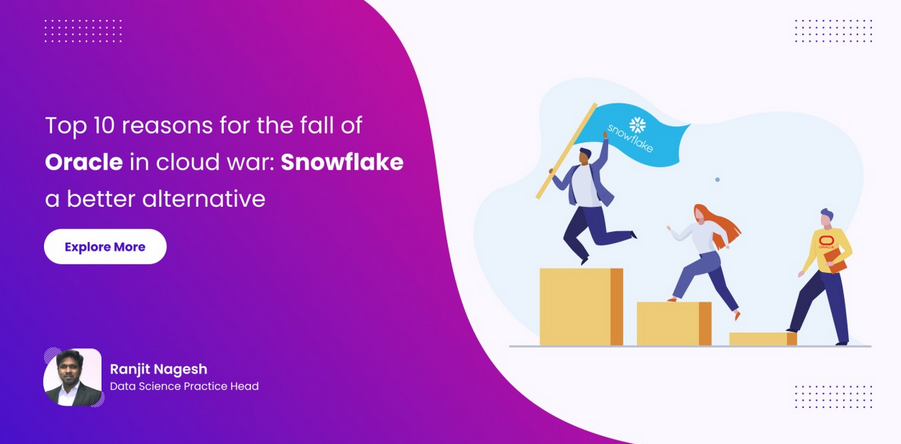
On the other hand, each layer is only the logical organization of code, with concerns organized and divided in a layered fashion. For example, you may create one or more projects in Visual Studio and organize your code into three layers. For example, a Razor Pages application depends on a business logic layer that depends on a data access layer. When you deploy that application, all these layers, including the database, are deployed together on the same server. This would be one tier and three layers. Of course, nowadays, chances are you have a cloud database somewhere, which adds a second tier to that architecture: the application tier (which still has three layers) and database tier.Now that we’ve discussed layers and tiers, let’s look at a layer versus an assembly.
What is an assembly?
Assemblies are commonly compiled into .dll or .exe files; you can compile and consume them directly. In most cases, each project of a Visual Studio solution gets compiled into an assembly. You can also deploy them as NuGet packages and consume them from nuget.org or a custom NuGet repository of your choosing. But there is no one-to-one relationship between a layer and an assembly or a tier and an assembly; assemblies are only consumable units of compiled code: a library or a program.Moreover, you do not need to split your layers into different assemblies; you can have your three layers residing in the same assembly. It can be easier to create undesirable coupling this way, with all of the code being in the same project, but it is a viable option with some rigor, rules, and conventions. Moving each layer to an assembly does not necessarily improve the application; the code inside each layer or assembly can become mixed up and coupled with other system parts.Don’t get me wrong: you can create an assembly per layer; I even encourage you to do so in most cases, but doing so does not mean the layers are not tightly coupled. A layer is simply a logical unit of organization, so each contributor’s responsibility is to ensure the layer’s code stays healthy.Furthermore, having multiple assemblies let us deploy them to one or more machines, potentially different machines, leading to multiple tiers.Let’s now look at the responsibilities of the most common layers.
Responsibilities of the common layers
In this section, we explore the most commonly used layers in more depth. We do not dig too deep into each one, but the overview should help you understand the essential ideas behind layering.
Presentation
The presentation layer is probably the easiest layer to understand because it is the only one we can see: the user interface. However, the presentation layer can also be the data contracts in case of a REST, OData, GraphQL, or other types of web service. The presentation layer is what the user uses to access your program. As another example, a CLI program can be a presentation layer. You write commands in a terminal, and the CLI dispatches them to its domain layer, executing the required business logic.The key to a maintainable presentation layer is to keep it as focused on displaying the user interface as possible with as little business logic as possible.Next, we look at the domain layer to see where these calls go.